Routing
We do not have to create a new file for every new page we add to our blog we can bundle to the whole frontend into one index file via routing. Here we will use the superglobal $_SERVER and its PATHINFO index to create individual paths and create all the pages within if/else statements like so:
<?php
session_start();
require("../src/init.php");
$pathInfo = $_SERVER["PATH_INFO"];
if($pathInfo == "/index") {
include("../views/layout/header.php");
include("../views/layout/nav.php");
include("../views/layout/footer.php");
// === BLOG ===
}elseif($pathInfo == "/blog") {
if(isset($_POST["comment"])){
header("location:blog?blogpost=" . $_GET["blogpost"]);
}
include("../views/layout/header.php");
include("../views/layout/nav.php");
//Integration Kategorieheader
include("../views/layout/categoryheader.php");
//Kategorie Links
$catcontroller = $container->make("catcontroller");
$catcontroller->category();
//Integration Kategoriefooter
include("../views/layout/categoryfooter.php");
//Postcontainer Header
include("../views/layout/postcontainerheader.php");
// Integration des Paginationmodel
include("../views/blog/pagination.php");
//Integration Paginationlayout oben
include("../views/layout/pagination.php");
//Integration der Postsvorschau
$postscontroller = $container->make("postscontroller");
$postscontroller->index($x, $postsProSeite);
//Integration KategorieBeschreibung + Postpreview
include("../views/blog/categorydetail.php");
//Integration Paginationlayout unten
include("../views/layout/pagination.php");
//Integration Blogpost
include("../views/blog/blogpost.php");
//Integration Hashtags Vorschau
include("../views/blog/hashtagspreview.php");
//Postcontainer Footer
include("../views/layout/postcontainerfooter.php");
//Integration Calendar
include("../views/layout/calendar.php");
//Integration Hashtags
include("../views/layout/hashtagsheader.php");
$hashcontroller = $container->make("hashcontroller");
$hashcontroller->all();
include("../views/layout/hashtagsfooter.php");
include("../views/layout/footer.php");
}elseif( $pathInfo == "/kontakt") {
include("../views/layout/header.php");
include("../views/layout/nav.php");
include("../views/layout/footer.php");
}elseif( $pathInfo == "/faq") {
include("../views/layout/header.php");
include("../views/layout/nav.php");
include("../views/layout/footer.php");
}elseif( $pathInfo == "/donate") {
include("../views/layout/header.php");
include("../views/layout/nav.php");
include("../views/layout/footer.php");
// === LOGIN ===
}elseif( $pathInfo == "/a-login") {
//session_regenerate_id(true);
include("../views/layout/header.php");
include("../views/layout/nav.php");
$logincontroller = $container->make("logincontroller");
$logincontroller->login();
include("../views/layout/footer.php");
}elseif($pathInfo == "/dashboard"){
session_regenerate_id(true);
include("../views/layout/header.php");
include("../views/layout/nav.php");
$logincontroller = $container->make("logincontroller");
$logincontroller->dashboard();
include("../views/layout/footer.php");
}elseif($pathInfo == "/logout"){
header("location: a-login");
include("../views/layout/header.php");
include("../views/layout/nav.php");
$logincontroller = $container->make("logincontroller");
$logincontroller->logout();
include("../views/layout/footer.php");
}
?>
Object-Oriented Programming in PHP Example: Category Widget using the Microblog Framework |
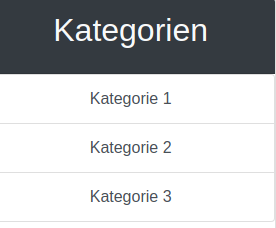
I’m now going to show how to create this widget using my framework
$catcontroller = $container->make("catcontroller");
$catcontroller->category();
In the last step we will enclose the widget with its header and footer. I nthis case the footer is only a closing tag, because the widget only has a header and body.
include("../views/layout/categoryheader.php");
//Kategorie Links
$catcontroller = $container->make("catcontroller");
$catcontroller->category();
//Integration Kategoriefooter
include("../views/layout/categoryfooter.php");
Download this project
You can download this project under the following Link:
https://drive.google.com/file/d/1XTFG_xxAGHyi0ITDc0HBs0WWakeYHUQe/view?usp=sharing
Admin & Database Login:
Admin: testadmin
Password: MPDqTMjWyWlGX0FD